How to build Bluetooth Socket
Requirements:
- Arduino
- Bluetooth Module: HC-06
- Android device
Arduino code
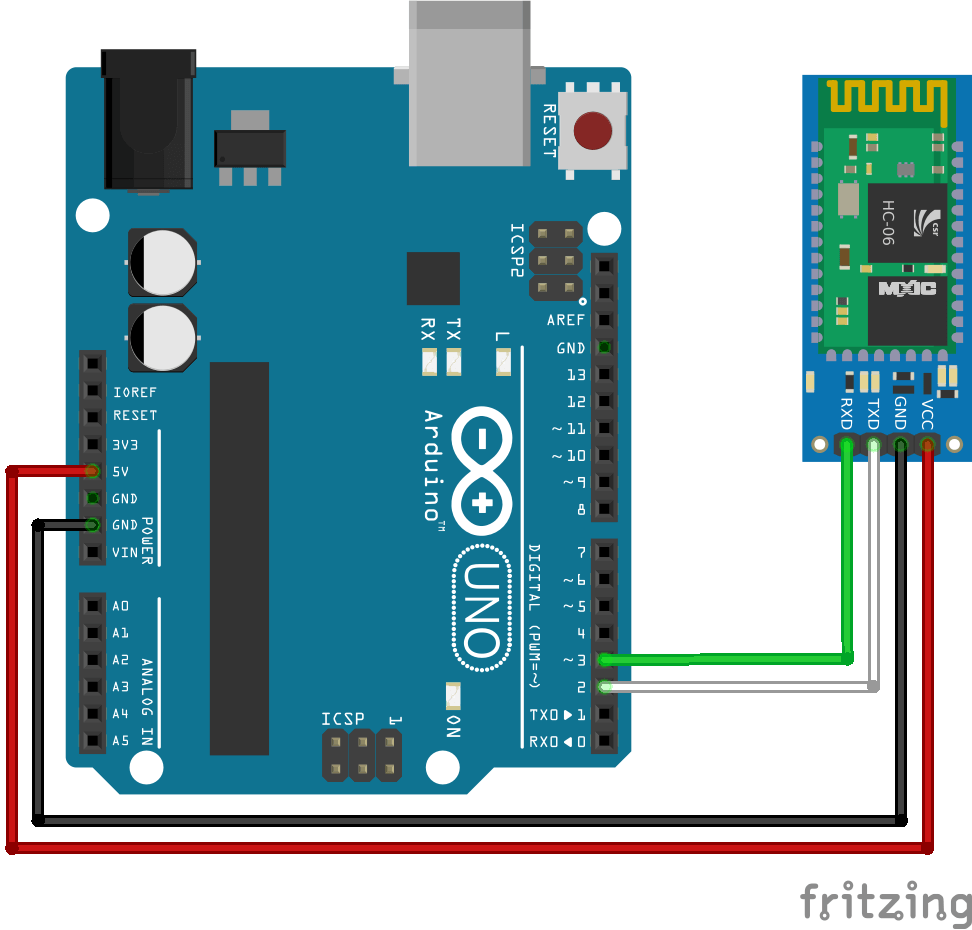
- Connect Arduino with HC06 module as in schematics above
- Upload following code into Arduino board
This code listens for any symbols received into serial terminal. Once it receives "0" symbol (48 from ASCII table), it must return English alphabet letters one by one (A-Z, 65 to 90 from ASCII):
/* BLESocketSketch.ino */ #include <SoftwareSerial.h> SoftwareSerial hc06(2, 3); byte receivedData; void setup() { hc06.begin(9600); // password is 0000 } void loop() { // check if HC06 is available if (hc06.available()) { // if text arrived from Bluetooth HC06 serial... receivedData = hc06.read(); // if symbol is "0" (ASCII) if (receivedData == 48) { // send alphabet for (int i = 65; i <= 90; i++) { hc06.print((char)i); delay(50); } } } delay(200); }
Android code
Instructions outline
- Define necessary Bluetooth permissions.
- Declare physical Mac address of Bluetooth device to connect.
- Get a handle to the default local
BluetoothAdapter
. Connect to remote device.
- Initialize
BluetoothSocket
for communication.
- Send "0" symbol to the socket.
- Receive input stream from Bluetooth socket.
- Don't forget to close
BluetoothSocket
after completion.
- Display results with
Logcat
of Android.
Step-by-step
Step 1. Define necessary Bluetooth permissions in Manifest.
<!-- AndroidManifest.xml -->
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN"/>
Step 2. Declare physical Mac address of Bluetooth device to connect.
/* MainActivity.java */
// for boards with bluetooth, use this UUID. For another mobile phone, use different
static final UUID mUUID = UUID.fromString("00001101-0000-1000-8000-00805F9B34FB");
// define mac address of device here (use your own mac address here)
private static final String arduinoMacAddress = "98:D3:32:70:A9:94";
You can download use this app from Play Store to get Mac address of Bluetooth device:
Step 3. Get a handle to the default local BluetoothAdapter
. Connect to remote device. Android Logcat command-line tool is useful to print outputs for debugging.
/* MainActivity.java */
// get default adapter and print paired devices
BluetoothAdapter btAdapter = BluetoothAdapter.getDefaultAdapter();
System.out.println(btAdapter.getBondedDevices());
// connect to the bluetooth with known mac address and print its name
BluetoothDevice hc06 = btAdapter.getRemoteDevice(arduinoMacAddress);
System.out.println(hc06.getName());
Step 4. Initialize Bluetooth socket for communication.
/* MainActivity.java */
// initialize Bluetooth socket for communication
BluetoothSocket btSocket = null;
int counter = 0;
do {
try {
// create Bluetooth socket
btSocket = hc06.createRfcommSocketToServiceRecord(mUUID);
System.out.println(btSocket);
// start connection
btSocket.connect();
System.out.println(btSocket.isConnected());
} catch (IOException e) {
e.printStackTrace();
}
counter++;
} while (!btSocket.isConnected() && counter < 3); // give 3 attempts
Step 5. Send "0" symbol to the socket.
/* MainActivity.java */
try {
// send char "0" on ASCII
OutputStream outputStream = btSocket.getOutputStream();
outputStream.write(48);
} catch (IOException e) {
e.printStackTrace();
}
Step 6. Receive input stream from Bluetooth socket.
/* MainActivity.java */
InputStream inputStream = null;
try {
// initialize and clear the input stream from HC06
inputStream = btSocket.getInputStream();
inputStream.skip(inputStream.available());
// convert received input bytes to alphabet
for (int k = 0; k < 26; k++) {
byte b = (byte) inputStream.read();
System.out.println((char) b); //System.out.println((char) b);
}
} catch (IOException e) {
e.printStackTrace();
}
Step 7. Don't forget to close the Bluetooth socket after completion.
/* MainActivity.java */
try {
// close Bluetooth socket
btSocket.close();
System.out.println(btSocket.isConnected());
} catch (IOException e) {
e.printStackTrace();
}
Step 8. Done! If you are successful here, you must be able to receive English alphabet letters and see them in Logcat of Android.