Building simple Bluetooth scanner
Demo
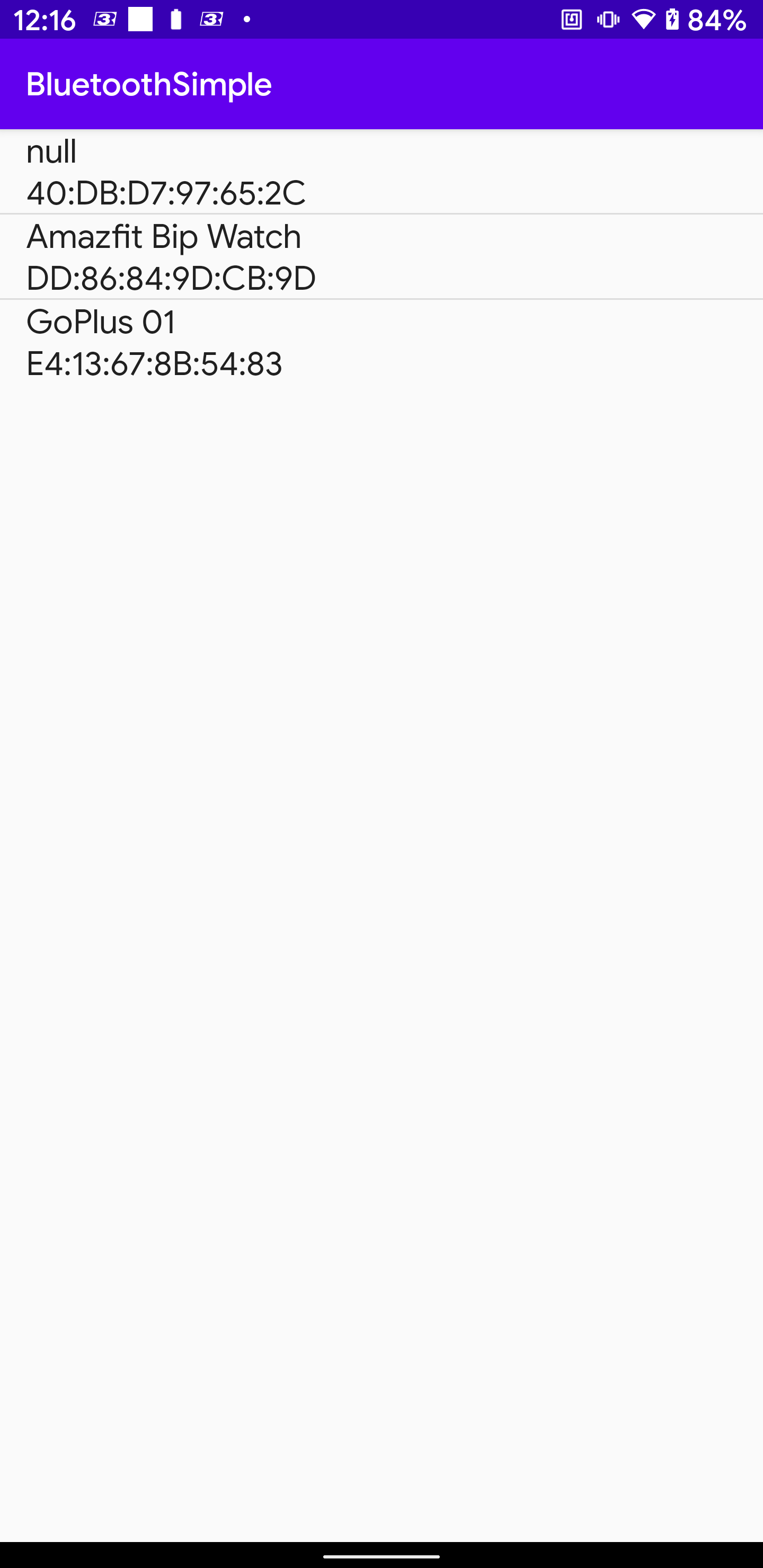
Overview
- Declare permissions in
Manifest
.
- Request
permissions
at runtime.
- Get a handle to the default local
BluetoothAdapter
.
- Start the remote device discovery process and register
BroadcastReceiver
.
- Get Bluetooth devices from
BroadcastReceiver
.
- Unregister
BroadcastReceiver
.
How to build
Step 1. Declare permissions in Manifest.
<!-- AndroidManifest.xml -->
<manifest ...">
...
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN" />
<!-- If your app targets Android 9 or lower, you can declare
ACCESS_COARSE_LOCATION instead. -->
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
...
</manifest>
Step 2. Request permissions at runtime.
/* MainActivity.java */
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
...
// check for permissions
if (checkSelfPermission(Manifest.permission.ACCESS_FINE_LOCATION) == PackageManager.PERMISSION_DENIED) {
String[] permission = {Manifest.permission.ACCESS_FINE_LOCATION};
requestPermissions(permission, PERMISSION_CODE);
} else {
checkBluetoothAdapter();
}
...
}
Step 3. Get a handle to the default local BluetoothAdapter
.
/* MainActivity.java */
private void checkBluetoothAdapter() {
mBluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
if (mBluetoothAdapter == null) {
Toast.makeText(this, "Device does not support Bluetooth", Toast.LENGTH_SHORT).show();
} else {
if (!mBluetoothAdapter.isEnabled()) {
Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(enableBtIntent, REQUEST_ENABLE_BT);
} else {
startBroadcastIntent();
}
}
}
Step 4. Start the remote device discovery process and register BroadcastReceiver
.
/* MainActivity.java */
public void startBroadcastIntent() {
// start discovery
mBluetoothAdapter.startDiscovery();
// register broadcast receiver and listen to specific "ACTION_FOUND" request
IntentFilter filter = new IntentFilter();
filter.addAction(BluetoothDevice.ACTION_FOUND);
this.registerReceiver(receiver, filter);
}
Step 5. Get Bluetooth devices from BroadcastReceiver
.
/* MainActivity.java */
private final BroadcastReceiver receiver = new BroadcastReceiver() {
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
// if remote bluetooth device found
if (BluetoothDevice.ACTION_FOUND.equals(action)) {
// receive related contents of new bluetooth device
BluetoothDevice device = intent.getParcelableExtra(BluetoothDevice.EXTRA_DEVICE);
if (device != null) {
// device name and MAC address
String deviceName = device.getName();
String deviceHardwareAddress = device.getAddress(); // MAC address
Log.d("BTDevice",deviceName + deviceHardwareAddress);
// add this info to list
mDeviceList.add(device.getName() + "\n" + device.getAddress());
// set contents into ListView
listView.setAdapter(new ArrayAdapter<>(context,
android.R.layout.simple_list_item_1, mDeviceList));
}
}
}
};
Step 6. Unregister BroadcastReceiver
.
/* MainActivity.java */
@Override
protected void onDestroy() {
super.onDestroy();
// unregister broadcast receiver
unregisterReceiver(receiver);
}