Week 0. Java: basics.
Credits: Ali
Classes and objects
Definitions
Java is object-oriented programming language. It means that you can represent any entities in terms of objects.
Class is a prototype, which describes what it has and how it behaves. Object, in turn, is a specific instance of a class. For example, we can model person as a class with name and age attributes, and give it specific functions to represent its behavior.
Classes have fields and methods.
Example
Main class
public class Main { public static void main(String[] args) { // create object john with name "John" and age 19 Person john = new Person("John", 19); System.out.println("Name: " + john.getName()); System.out.println("Age: " + john.getAge()); // create another object alex with name "Alex" and age 20 Person alex = new Person("Alex", 20); // set best friend of John to Alex john.addBestFriend(alex); // print best friend of John john.printBestFriend(); } }
Person class
/* Person class Has name, age and best friend fields Best friend field is a reference to another object of Person class */ public class Person { // class fields private String name; private int age; private Person bestFriend; // constructor method public Person(String name, int age) { this.name = name; this.age = age; } // name getter public String getName() { return this.name; } // age getter public int getAge() { return this.age; } // custom class methods public void addBestFriend(Person p) { this.bestFriend = p; } public void printBestFriend() { System.out.println("The best friend of " + this.name + " is " + this.bestFriend.getName()); } }
Properties
Inheritance
Classes can inherit fields and methods from another class through inheritance mechanism. To inherit from a class, you need to use extends keyword.
Example
Main class
public class Main { public static void main(String[] args) { // create Animal object Animal a = new Animal(4); // call eat() and sound() methods of this object a.eat(); a.sound(); // create Dog object Dog ralph = new Dog(5); // call eat() and sound() methods of ralph ralph.eat(); ralph.sound(); } }
Animal class
/* Animal class Has only age field */ public class Animal { // class fields private int age; // constructor method public Animal(int age) { this.age = age; } // age getter public int getAge() { return this.age; } // custom class methods public void eat() { System.out.println("Default eating"); } public void sound() { System.out.println("Default animal sound"); } }
Dog class
/* Dog class Inherits from Animal class Overrides eat() and sound() methods */ public class Dog extends Animal { // constructor method public Dog(int age) { super(age); } // overriden methods public void eat() { System.out.println("Dog eating, e.g. omnomnom"); } public void sound() { System.out.println("Bark bark!"); } }
Variables
private
, protected
and public
:
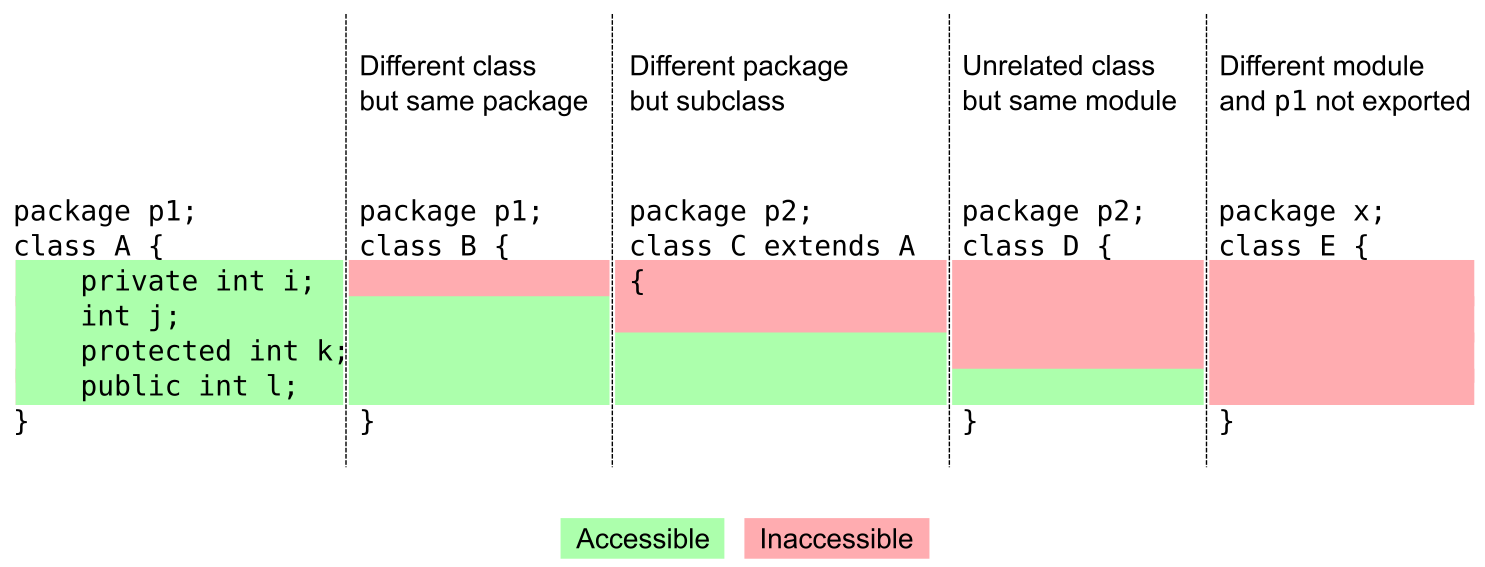
Explanations
- A private member (
i
) is only accessible within the same class as it is declared.
- A member with no access modifier (
j
) is only accessible within classes in the same package.
- A protected member (
k
) is accessible within all classes in the same package and within subclasses in other packages.
- A public member (
l
) is accessible to all classes (unless it resides in a module that does not export the package it is declared in).
final
and static final
For final, it can be assigned different values at runtime when initialized:
Class Test {
public final int a;
}
Test t1 = new Test();
t1.a = 10;
Test t2 = new Test();
t2.a = 20; //fixed
Thus each instance has different value of field a
.
For static final, all instances share the same value, and can’t be altered after first initialized:
Class TestStatic {
public static final int a;
}
Test t1 = new Test();
t1.a = 10;
Test t2 = new Test();
t1.a = 20; // ERROR, CAN'T BE ALTERED AFTER THE FIRST INITIALIZATION.
Further reading
Object-oriented programming in Java with game example
http://gamecodeschool.com/java/understanding-oop-for-coding-java-games/
Java tutorial on w3schools
https://www.w3schools.com/java/